声控聊天GPT: 利用JavaScript实现麦克风输入
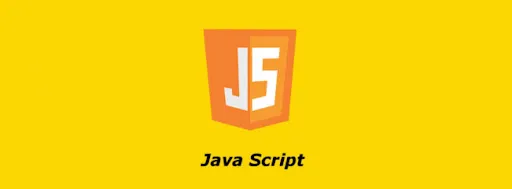
简介
在这篇博客文章中,我们将探讨如何使用JavaScript从麦克风捕获语音输入,并将该输入作为提示发送到OpenAI的ChatGPT API。这种创新方法允许用户通过语音命令与人工智能互动,提升可访问性和用户体验。
先决条件
在我们开始之前,请确保您拥有以下内容:
- HTML、CSS和JavaScript的基本知识。
- 一个来自OpenAI的API密钥,用于访问ChatGPT模型。
步骤1:获取您的API密钥
- 注册/登录到OpenAI:访问OpenAI网站,如果你还没有账号,请先创建一个。
- 获取您的API密钥:转到API部分并生成您的API密钥。请保管好这个密钥,因为它将用于身份验证。
第二步:设置您的项目
为您的项目创建一个新目录,并创建两个文件:index.html 和 app.js。
目录结构
/voice-chatgpt
├── index.html
└── app.js
步骤3:创建HTML结构
在index.html中设置一个简单的HTML结构,使用户可以开始和停止录制他们的语音输入。
HTML 代码(index.html)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset=UTF-8 <meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Voice Activated ChatGPT</title>
<style>
body {
font-family: Arial, sans-serif;
margin: 20px;
}
#response {
margin-top: 10px;
border: 1px solid #ccc;
padding: 10px;
min-height: 50px;
}
.button {
padding: 10px 20px;
margin: 10px;
cursor: pointer;
}
</style>
</head>
<body>
<h1>Voice-Activated Chat with ChatGPT</h1>
<button id="start-button" class="button">Start Recording</button>
<button id="stop-button" class="button" disabled>Stop Recording</button>
<div id="response"></div>
<script src="app.js"></script>
</body>
</html>
第四步:实现JavaScript功能.
在app.js文件中,我们将实现从麦克风录制音频的逻辑,使用Web Speech API将其转换为文本,然后将文本输入发送到ChatGPT API。
JavaScript 代码(app.js)
const apiKey = 'YOUR_API_KEY'; // Replace with your actual OpenAI API key
const startButton = document.getElementById('start-button');
const stopButton = document.getElementById('stop-button');
const responseDiv = document.getElementById('response');
let recognition;
// Initialize Speech Recognition
if ('webkitSpeechRecognition' in window) {
recognition = new webkitSpeechRecognition();
recognition.continuous = false; // Stop automatically after the first result
recognition.interimResults = false; // We want only final results
recognition.onresult = async (event) => {
const userMessage = event.results[0][0].transcript;
responseDiv.innerHTML = "You said: " + userMessage;
await sendToChatGPT(userMessage);
};
recognition.onerror = (event) => {
console.error('Error occurred in recognition: ' + event.error);
responseDiv.innerHTML = "Error occurred while recognizing speech.";
};
} else {
alert('Your browser does not support speech recognition. Please use Chrome or Edge.');
}
startButton.addEventListener('click', () => {
recognition.start();
startButton.disabled = true;
stopButton.disabled = false;
});
stopButton.addEventListener('click', () => {
recognition.stop();
startButton.disabled = false;
stopButton.disabled = true;
});
async function sendToChatGPT(userMessage) {
responseDiv.innerHTML += "<br/>Loading response...";
try {
const response = await fetch('https://api.openai.com/v1/chat/completions', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${apiKey}`
},
body: JSON.stringify({
model: "gpt-3.5-turbo",
messages: [{ role: "user", content: userMessage }]
})
});
const data = await response.json();
const botReply = data.choices[0].message.content;
responseDiv.innerHTML += `<br/>ChatGPT says: ${botReply}`; // Display the bot's response
} catch (error) {
console.error('Error:', error);
responseDiv.innerHTML += "<br/>Error occurred while fetching response.";
}
}
步骤5:运行您的应用程序
- 插入您的API密钥:打开app.js文件,将YOUR_API_KEY替换为您的实际OpenAI API密钥。
- 打开index.html:在您的网络浏览器中启动index.html文件。
- 与ChatGPT互动:点击“开始录音”按钮开始捕捉您的语音输入。说出您的提示,然后点击“停止录音”。该应用程序将处理您的输入,并显示来自ChatGPT的响应。
结论
在这篇博客文章中,我们成功地构建了一个声控应用程序,允许用户使用麦克风与ChatGPT互动。这个项目展示了结合JavaScript、Web Speech API和OpenAI的ChatGPT创造直观用户体验的强大力量。
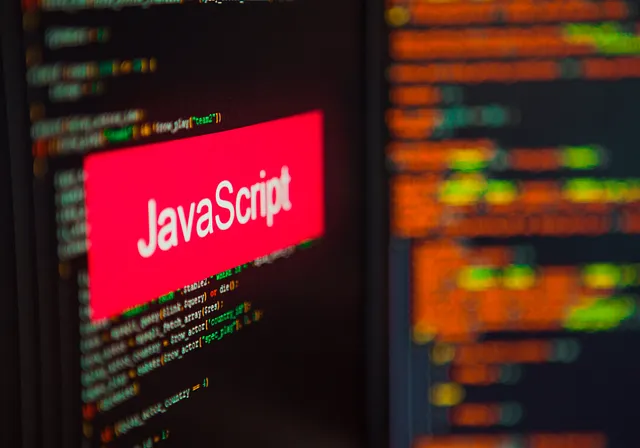