使用Azure OpenAI服务API - 通过函数调用进行流媒体处理
使用Azure OpenAI进行流式处理的功能调用
介绍
功能调用使您能够将模型(如GPT-4)与外部工具和系统进行链接。这对各种目的都是有益的,包括用额外功能增强人工智能助理,并在您的应用程序和模型之间创建稳健的集成。
函数调用在聊天完成 API、助手 API 和批处理 API 中均受支持。然而,这份简短指南侧重于使用聊天完成 API 进行函数调用,并使用 Azure OpenAI 服务进行流式处理。
单个工具/功能调用示例
在这个指南中,我们创建了一个样本函数,用来调用雅虎金融API,来检索给定股票代码的股票价格。
在高层次上,使用函数可以分为三个步骤:
- 使用聊天完成API与您的函数和用户的输入。
- 利用模型的响应来调用您的API或函数。
- 再次调用聊天完成API,将您的函数的响应加入其中以获取最终的回复。
注意:创建Python虚拟环境或Conda环境超出本文档范围。请确保已安装所需的库使用pip.
注意:确保提供Azure OpenAI端点、API密钥、API版本和模型部署名称。
有打印语句和注释的打印语句可以帮助理解代码。如果需要深入了解,取消注释这些打印语句。例如,分块中的增量是什么样子。
import os
import openai
from dotenv import load_dotenv
import json
import yfinance as yf
from datetime import datetime
# load environment variable.
load_dotenv()
# Load OpenAI variables. Make sure to provide those variables as per your environment.
azure_endpoint: str = os.environ["AZURE_OPENAI_ENDPOINT"] #PLACEHOLDER FOR YOUR AZURE OPENAI ENDPOINT
azure_openai_api_key: str = os.environ["AZURE_OPENAI_API_KEY"] #PLACEHOLDER FOR YOUR AZURE OPENAI KEY
azure_openai_api_version: str = os.environ["AZURE_OPENAI_API_VERSION"] # API VERSION PLACE HOLDER
azure_deployment: str = os.environ["OPENAI_DEPLOYMENT_NAME_GPT4o"] # AZURE OPENAI DEPLOYMENT NAME
# Define the function for the model
tools = [
{
"type": "function",
"function": {
"name": "get_stock_price",
"description": "Retrieve lowest and highest stock price for a given stock symbol and date",
"parameters": {
"type": "object",
"properties": {
"symbol": {
"type": "string",
"description": "Stock symbol, for example MSFT for Microsoft"
}
},
"required": ["symbol"],
},
}
}
]
# Initialize Azure OpenAI client with key-based authentication
client = openai.AzureOpenAI(
azure_endpoint = azure_endpoint,
api_key = azure_openai_api_key,
api_version = azure_openai_api_version,
)
# create a funtion
def get_stock_price(symbol):
print(f"Getting stock price for {symbol}")
ticker = yf.Ticker(symbol)
info = ticker.info
date = datetime.today().strftime('%Y-%m-%d')
return json.dumps({
"symbol": symbol,
"date": date,
"current price": info['currentPrice'],
"low price": info['dayLow'],
"high price": info['dayHigh'],
"opening price": info['open'],
"link": f"https://finance.yahoo.com/quote/{symbol}/"
}
)
# initial user message
messages = []
tool_call_accumulator = ""
messages.append({"role": "user", "content": "what is the price of tesla stock"}) # single function call
# First API call: Ask the model to use the function
response = client.chat.completions.create(
model= azure_deployment,
messages=messages,
tools=tools,
tool_choice="auto",
stream=True # this time, we set stream=True
)
# process the model
for chunk in response:
if len(chunk.choices) > 0:
delta = chunk.choices[0].delta
#print(f"delta: {delta}") # print delta from the chunk for learning
if delta.tool_calls:
if(delta.tool_calls[0].function.name):
tool_name = chunk.choices[0].delta.tool_calls[0].function.name
tool_id = chunk.choices[0].delta.tool_calls[0].id
messages.append(delta)
if(chunk.choices[0].delta.tool_calls[0].function.arguments):
tool_call_accumulator+=delta.tool_calls[0].function.arguments
# print function related outputs
print(f"tool_name:{tool_name}")
print(f"tool_id:{tool_id}")
print(f"tool_call_accumulator:{tool_call_accumulator}")
# handle the function call
if tool_name == "get_stock_price":
function_args=json.loads(tool_call_accumulator)
#print(function_args.get("symbol"))
stock_price_data = get_stock_price(symbol=function_args.get("symbol"))
#print(f"stock_price_data: {stock_price_data}")
messages.append({
"tool_call_id": tool_id,
"role": "tool",
"name": tool_name,
"content": stock_price_data,
})
#print(f"messages: {messages}")
print("=============================================")
# second API call: Get the final response with stream
response = client.chat.completions.create(
model= azure_deployment,
messages=messages,
temperature=0.7,
stream=True # this time, we set stream=True
)
for chunk in response:
if len(chunk.choices) > 0:
delta = chunk.choices[0].delta
#print(f"delta: {delta}")
if delta.content:
print(delta.content, end="", flush=True)
输出:
PS C:\Development\openai\chatgpt-demo> & C:/Users/muafzal/Miniconda3/envs/.conda39/python.exe c:/Development/openai/chatgpt-demo/Azure_OpenAI_FunctionCall_With_Stream.py
tool_name:get_stock_price
tool_id:call_grJz8BFcBcfIQD8HAdDuuQ6U
tool_call_accumulator:{"symbol":"TSLA"}
Getting stock price for TSLA
=============================================
As of the latest data on October 6, 2024, the price of Tesla (TSLA) stock is $250.08. Here are some additional details:
- **Low Price:** $244.58
- **High Price:** $250.96
- **Opening Price:** $246.7
For more information, you can visit [Tesla on Yahoo Finance](https://finance.yahoo.com/quote/TSLA/).
PS C:\Development\openai\chatgpt-demo>
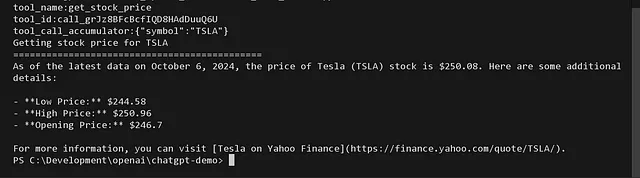
结论
Azure OpenAI 函数调用增强了 AI 助手的体验。它提供了动态数据检索和响应。总的来说,OpenAI 函数调用弥合了静态语言模型响应和动态、可操作、数据驱动的结果之间的差距。