LangChain - 生成式人工智能 - 概览
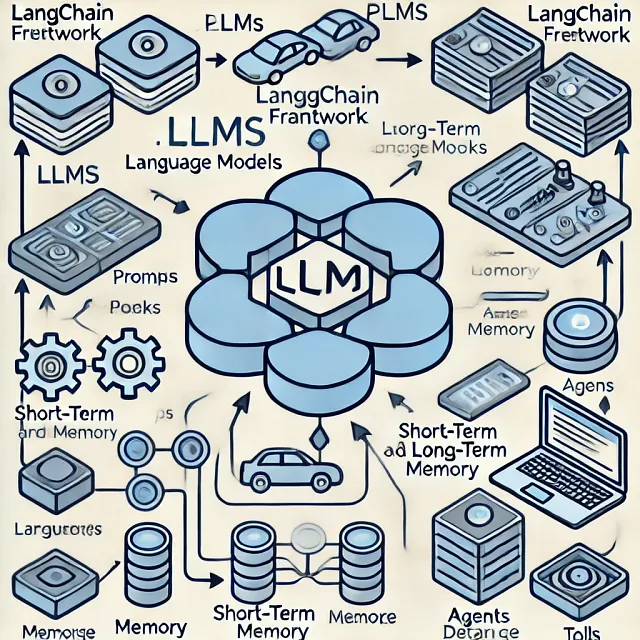
它是一个框架,允许开发人员使用LLMs创建应用程序。它将外部组件与LLMs结合起来。它还可以为现有的LLMs添加上下文和记忆,帮助它们完成更复杂的任务。特点包括一个集中的开发环境,基于模块的方法,以及比较不同提示和基础模型的能力。它可以与其他工具和框架集成,包括高性能的NoSQL数据库Couchbase。
如何构建应用程序
让我们开始构建Langchain的简单原型或用例。
- 通用- 单链是最简单的链条。 它有两个输入部分:-
- 输入提示
- LLM的名称
用于生成文本。让我们建立一个基本的链——创建一个提示并得到一个预测。
import os
from langchain.prompts import PromptTemplate
os.environ["OPENAI_API_KEY"] = "..."
prompt = PromptTemplate(
input_variables=["product"],
template="What is a good name for a company that makes {product}?",
)
print(prompt.format(product="podcast player"))
from langchain.llms import OpenAI
from langchain.chains import LLMChain
llm = OpenAI(
model_name="text-davinci-003", # default model
temperature=0.9) #temperature dictates how whacky the output should be
llmchain = LLMChain(llm=llm, prompt=prompt)
llmchain.run("podcast player")
from langchain.chat_models import ChatOpenAI
chatopenai = ChatOpenAI(
model_name="gpt-3.5-turbo")
llmchain_chat = LLMChain(llm=chatopenai, prompt=prompt)
llmchain_chat.run("podcast player")
通用链用作实用链的构建模块。
- 效用:它由多个链组成,可帮助解决问题。一个示例是PalChain。 PAL代表Programme Aided Language Model。
它读取一个数学问题,生成程序来解决它,并将解决步骤转移到运行时,如Python解释器。
再次,它分为两部分:
- 首先,它使用一个通用的LLMChain来理解查询。
- 其次,Python REPL 解决了由LLM输出的函数/程序。
从langchain.chains导入PALChain palchain = PALChain.from_math_prompt(llm=llm, verbose=True) palchain.run("如果我的年龄是我爸爸年龄的一半,他明年将满60岁,那么我现在多大?")
# OUTPUT
# > Entering new PALChain chain...
# def solution():
# """If my age is half of my dad's age and he is going to be 60 next year, what is my current age?"""
# dad_age_next_year = 60
# dad_age_now = dad_age_next_year - 1
# my_age_now = dad_age_now / 2
# result = my_age_now
# return result
#
# > Finished chain.
# '29.5'
它有一个默认提示传递。
print(palchain.prompt.template)
构建链条 我们已经设定了自己去构建链条。我们需要弄清楚如何将一个链条的输出传递给另一个链条作为输入。我们可以使用SimpleSequentialChain。链条的一个用例是改进OpenAI—ChatGPT,因为它受到用于构建模型的信息的时间限制。
我们有一个类似于LLMs的概念:代理人。代理人可以访问LLMs和一套工具,如Google搜索,Python REPL,数学计算器,天气API等。最常见的代理人之一是zero-shot-react-description。这个工具使用ReAct(Reason+Act)框架来选择最适用的工具。我们需要初始化代理人并传递它所需的工具和LLM。例如,我们正在使用pal-math。我们将传递与初始化之前使用的相同的LLM。
from langchain.agents import initialize_agent
from langchain.agents import AgentType
from langchain.agents import load_tools
llm = OpenAI(temperature=0)
tools = load_tools(["pal-math"], llm=llm)
agent = initialize_agent(tools,
llm,
agent=AgentType.ZERO_SHOT_REACT_DESCRIPTION,
verbose=True)
让我们在上面相同的示例上进行测试:
agent.run("If my age is half of my dad's age and he is going to be 60 next year, what is my current age?")
# OUTPUT
# > Entering new AgentExecutor chain...
# I need to figure out my dad's current age and then divide it by two.
# Action: PAL-MATH
# Action Input: What is my dad's current age if he is going to be 60 next year?
# Observation: 59
# Thought: I now know my dad's current age, so I can divide it by two to get my age.
# Action: Divide 59 by 2
# Action Input: 59/2
# Observation: Divide 59 by 2 is not a valid tool, try another one.
# Thought: I can use PAL-MATH to divide 59 by 2.
# Action: PAL-MATH
# Action Input: Divide 59 by 2
# Observation: 29.5
# Thought: I now know the final answer.
# Final Answer: My current age is 29.5 years old.
# > Finished chain.
# 'My current age is 29.5 years old.
它执行三个步骤中的一个来完成任务。
- 观察
- 思想
- 行动
这主要是由于ReAct框架和相关提示所致,代理正在使用:
print(agent.agent.llm_chain.prompt.template)
# OUTPUT
# Answer the following questions as best you can. You have access to the following tools:
# PAL-MATH: A language model that is really good at solving complex word math problems. Input should be a fully worded hard word math problem.
# Use the following format:
# Question: the input question you must answer
# Thought: you should always think about what to do
# Action: the action to take, should be one of [PAL-MATH]
# Action Input: the input to the action
# Observation: the result of the action
# ... (this Thought/Action/Action Input/Observation can repeat N times)
# Thought: I now know the final answer
# Final Answer: the final answer to the original input question
# Begin!
# Question: {input}
# Thought:{agent_scratchpad}
代理提升是使用未知链的能力,在预定的LLMs /其他工具链调用中通常是不可能的。例如,OpenAI有过时的信息,因为它是使用截至2020年的数据构建的。
agent.run("我现在的年龄是我爸爸年龄的一半。明年他的年龄将会和黛米·摩尔一样。我现在几岁?")
这可以通过包含另一种工具来轻松解决 — 工具 = load_tools([“pal-math”, “serpapi”], llm=llm)。serpapi 用于回答关于当前事件的问题是很有用的。
使用案例1
另一个例子是播客 API。
tools = load_tools(["podcast-api"], llm=llm, listen_api_key="...")
agent = initialize_agent(tools,
llm,
agent=AgentType.ZERO_SHOT_REACT_DESCRIPTION,
verbose=True)
agent.run("Show me episodes for money saving tips.")
# OUTPUT
# > Entering new AgentExecutor chain...
# I should search for podcasts or episodes related to money saving
# Action: Podcast API
# Action Input: Money saving tips
# Observation: The API call returned 3 podcasts related to money saving tips: The Money Nerds, The Rachel Cruze Show, and The Martin Lewis Podcast. These podcasts offer valuable money saving tips and advice to help people take control of their finances and create a life they love.
# Thought: I now have some options to choose from
# Final Answer: The Money Nerds, The Rachel Cruze Show, and The Martin Lewis Podcast are great podcast options for money saving tips.
# > Finished chain.
它有两个部分。
- 第一部分涉及根据我们的输入指令创建API URL并发起API调用。
- 第二部分是关于总结回答。
使用案例2:将链条组合以创建一个年龄合适的礼物生成器。我们将根据之前关于年龄问题的使用案例创建我们自己的顺序链条。我们将把它们组合起来:
- 链#1:- 我们刚刚创建的代理,可以解决数学中的年龄问题。
- 链条#2:- 一种以一个人的年龄为基础并建议适合他们的礼物的LLM。
# Chain1 - solve math problem, get the age
chain_one = agent
# Chain2 - suggest age-appropriate gift
template = """You are a gift recommender. Given a person's age,\n
it is your job to suggest an appropriate gift for them.
Person Age:
{age}
Suggest gift:"""
prompt_template = PromptTemplate(input_variables=["age"], template=template)
chain_two = LLMChain(llm=llm, prompt=prompt_template)
我们可以使用SimpleSequentialChain将它们组合在一起。
from langchain.chains import SimpleSequentialChain
overall_chain = SimpleSequentialChain(
chains=[chain_one, chain_two],
verbose=True)
以下是再次运行的相同数学问题:
question = "If my age is half of my dad's age and he is going to be 60 next year, what is my current age?"
overall_chain.run(question)
# OUTPUT
# > Entering new SimpleSequentialChain chain...
# > Entering new AgentExecutor chain...
# I need to figure out my dad's current age and then divide it by two.
# Action: PAL-MATH
# Action Input: What is my dad's current age if he is going to be 60 next year?
# Observation: 59
# Thought: I now know my dad's current age, so I can divide it by two to get my age.
# Action: Divide 59 by 2
# Action Input: 59/2
# Observation: Divide 59 by 2 is not a valid tool, try another one.
# Thought: I need to use PAL-MATH to divide 59 by 2.
# Action: PAL-MATH
# Action Input: Divide 59 by 2
# Observation: 29.5
# Thought: I now know the final answer.
# Final Answer: My current age is 29.5 years old.
# > Finished chain.
# My current age is 29.5 years old.
# Given your age, a great gift would be something that you can use and enjoy now like a nice bottle of wine, a luxury watch, a cookbook, or a gift card to a favorite store or restaurant. Or, you could get something that will last for years like a nice piece of jewelry or a quality leather wallet.
# > Finished chain.
我们还可以使用SimpleMemory将额外信息传递给第二个链。例如,让我们将预算作为输入变量添加进去。
template = """You are a gift recommender. Given a person's age,\n
it is your job to suggest an appropriate gift for them. If age is under 10,\n
the gift should cost no more than {budget} otherwise it should cost atleast 10 times {budget}.
Person Age:
{output}
Suggest gift:"""
prompt_template = PromptTemplate(input_variables=["output", "budget"], template=template)
chain_two = LLMChain(llm=llm, prompt=prompt_template)
我们必须小心输出变量,这已经从年龄变了。
print(agent.agent.llm_chain.output_keys)
# OUTPUT
["output"]
SimpleSequentialChain 只能处理单个输入和单个输出。现在,我们要使用两个输入,再加上一个预算作为额外变量。因此,我们需要使用 SequentialChain,可以处理多个输入和输出。
overall_chain = SequentialChain(
input_variables=["input"],
memory=SimpleMemory(memories={"budget": "100 GBP"}),
chains=[agent, chain_two],
verbose=True)
我们需要注意第一个代理使用的输入变量名称,这可以通过检查代码找到。
print(agent.agent.llm_chain.prompt.template)
# OUTPUT
#Answer the following questions as best you can. You have access to the following tools:
#PAL-MATH: A language model that is really good at solving complex word math problems. Input should be a fully worded hard word math problem.
#Use the following format:
#Question: the input question you must answer
#Thought: you should always think about what to do
#Action: the action to take, should be one of [PAL-MATH]
#Action Input: the input to the action
#Observation: the result of the action
#... (this Thought/Action/Action Input/Observation can repeat N times)
#Thought: I now know the final answer
#Final Answer: the final answer to the original input question
#Begin!
#Question: {input}
#Thought:{agent_scratchpad}
最后,让我们用相同的提示来运行新链。
overall_chain.run("If my age is half of my dad's age and he is going to be 60 next year, what is my current age?")
# OUTPUT
#> Entering new SequentialChain chain...
#> Entering new AgentExecutor chain...
# I need to figure out my dad's current age and then divide it by two.
#Action: PAL-MATH
#Action Input: What is my dad's current age if he is going to be 60 next year?
#Observation: 59
#Thought: I now know my dad's current age, so I can divide it by two to get my age.
#Action: Divide 59 by 2
#Action Input: 59/2
#Observation: Divide 59 by 2 is not a valid tool, try another one.
#Thought: I can use PAL-MATH to divide 59 by 2.
#Action: PAL-MATH
#Action Input: Divide 59 by 2
#Observation: 29.5
#Thought: I now know the final answer.
#Final Answer: My current age is 29.5 years old.
#> Finished chain.
# For someone of your age, a good gift would be something that is both practical and meaningful. Consider something like a nice watch, a piece of jewelry, a nice leather bag, or a gift card to a favorite store or restaurant.\nIf you have a larger budget, you could consider something like a weekend getaway, a spa package, or a special experience.'}
#> Finished chain.
For someone of your age, a good gift would be something that is both practical and meaningful. Consider something like a nice watch, a piece of jewelry, a nice leather bag, or a gift card to a favorite store or restaurant.\nIf you have a larger budget, you could consider something like a weekend getaway, a spa package, or a special experience.'}
结论这是关于LangChain的概述,它可以用于使用LLMs构建应用程序。我们还分享了代理的概念以及它们的用途。关于使用LangChain改进应用程序的概念还有很多,比如如何优化内存,以便我们可以选择性地摘要对话内容。希望您阅读这篇文章时愉快。
参考资料[1] https://towardsdatascience.com/a-gentle-intro-to-chaining-llms-agents-and-utils-via-langchain-16cd385fca81