使用ChatGPT设计一个多智能体交易系统来进行决策
本指南通过利用ChatGPT作为每个代理人的中央决策工具,扩展了多代理人交易系统的设计。 ChatGPT不仅提升了每个代理人的表现,还通过先进的模拟和分析能力提供了动态、实时的见解。在这里,每个代理人的决策都基于ChatGPT的输出,使系统更加智能、适应性更强,并能处理复杂的市场情况。
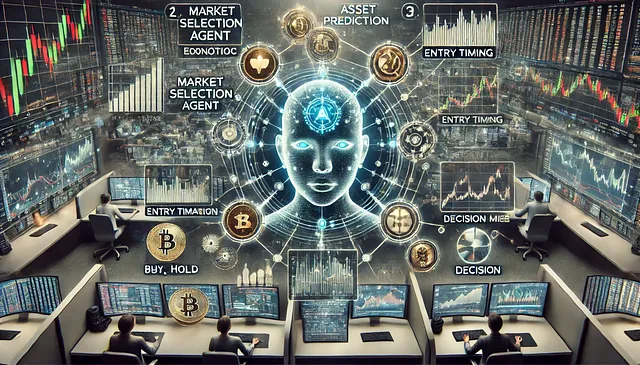
为什么ChatGPT是交易系统中决策的理想选择
1. 高级自然语言处理(NLP):ChatGPT 可以实时处理大量文本数据,如新闻文章、社交媒体帖子和财务报告。这种能力使其能够提取情感,检测市场趋势,并理解传统模型可能忽略的微妙信息。
2. 情景模拟与假设分析:ChatGPT可以模拟各种市场情景,让交易员根据经济因素、新闻事件或技术指标的假设性变化,预见潜在结果。这种能力对于完善策略和做出更明智的决策至关重要。
3. 持续学习与适应:ChatGPT 不断从新数据中学习,使其成为适应不断变化市场条件的强大工具。与静态模型不同,它随着数据的演变而发展,随着时间的推移增强其预测准确性。
4. 广泛的知识库:ChatGPT 可以访问包括历史数据、金融概念和技术分析技巧在内的庞大知识库。这种知识使其能够提供全面的建议,融入多重视角。
5. 实时分析和决策支持:ChatGPT能够生成实时响应,使其可以在需要时支持交易决策,这使其成为高频交易环境中时间至关重要的极佳工具。
系统概述,以ChatGPT作为核心决策者
在多主体交易系统中,每个代理人都使用ChatGPT进行所有关键决策。ChatGPT充当交互式顾问,提供实时见解,模拟结果,并根据最新数据改进交易策略。
- 市场选择代理:使用ChatGPT分析宏观经济因素,模拟潜在市场影响,选择最佳市场进入。
- 资产预测代理:依赖于ChatGPT进行资产表现预测分析,结合定量数据和定性情感。
- 输入定时代理:使用ChatGPT根据模拟技术分析和假设测试确定最佳进入点。
- 监控代理:通过ChatGPT持续监测资产表现,考虑实时数据、新闻事件和实时分析。
- 决策代理人:使用ChatGPT根据当前市场状况、绩效数据和风险评估决定何时买入、持有或卖出。
基于ChatGPT的决策实施细节
情景1:使用ChatGPT进行市场选择
市场选择代理人使用ChatGPT根据全面分析和模拟来做出市场决策。
为什么ChatGPT在这里有效:
- ChatGPT结合宏观经济数据和情绪分析,提供全面的市场视角。
- 它模拟了不同的情景来确定哪个市场提供了最好的机会,增强决策过程。
情景2:使用ChatGPT进行资产预测
资产预测代理依赖于ChatGPT根据绩效预测、情绪分析和模拟结果来选择最佳资产。
为什么在这里ChatGPT 是有效的?
- ChatGPT分析历史和当前数据,提供资产预测。
- 它结合了新闻情绪和市场趋势,提供了更加细致的资产选择过程。
情境3: 使用ChatGPT的输入时机
进场定时代理使用ChatGPT来识别最佳入市时间,通过模拟各种进场策略。
为什么ChatGPT在这里非常有效:
- ChatGPT 同时评估多个技术指标,提供全面的评估。
- 它模拟不同的入口点和策略来确定最佳方法。
情景4:使用ChatGPT进行监控和决策制定
监控和决策代理不断地与ChatGPT进行交互,分析实时数据并做出明智的买入、持有或卖出决策。
为什么 ChatGPT 在这里有效:
- ChatGPT提供动态、实时的反馈,随着市场条件变化调整其建议。
- 它不断分析技术指标、价格趋势和市场情绪,以完善其决策。
最终系统编排与ChatGPT
主要应用程序组织ChatGPT驱动的代理以创建完全由人工智能驱动的交易系统。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net.Http;
using System.Threading;
using System.Threading.Tasks;
using Newtonsoft.Json.Linq;
// ChatGPT Session Class - Placeholder for actual API integration with OpenAI or similar models
public class ChatGPTSession
{
// Method to simulate a ChatGPT response based on a query
public string Ask(string query)
{
// Replace this with actual ChatGPT API call
Console.WriteLine($"Query to ChatGPT: {query}");
// Simulated response; you should replace it with the actual API response handling
if (query.Contains("market"))
return "Crypto"; // Example: ChatGPT decides Crypto is the most favorable market
if (query.Contains("performance"))
return "0.8"; // Example: Performance score for an asset
if (query.Contains("good time"))
return "Yes, it's a good time to enter."; // Entry timing decision
if (query.Contains("Buy, Hold, or Sell"))
return "Sell"; // Example: ChatGPT suggests selling
return "No decision made"; // Fallback response
}
// Method to simulate sentiment analysis using ChatGPT
public double AnalyzeSentiment(string input)
{
// Replace this with actual ChatGPT sentiment analysis functionality
Console.WriteLine($"Sentiment analysis request: {input}");
return 0.7; // Example sentiment score
}
}
// Market Selection Agent
public class MarketSelectionAgent
{
private ChatGPTSession _chatGPT;
public MarketSelectionAgent()
{
_chatGPT = new ChatGPTSession();
}
public string SelectMarket()
{
// Fetch data for analysis
double inflationRate = GetInflationRate();
double interestRate = GetInterestRate();
double sentimentScore = AnalyzeSentiment();
// Ask ChatGPT to decide the best market based on economic conditions
string decision = _chatGPT.Ask($"Given an inflation rate of {inflationRate}, interest rate of {interestRate}, and market sentiment score of {sentimentScore}, which market (Stock, Crypto, Gold) should we enter?");
return decision;
}
private double GetInflationRate()
{
// Placeholder: Fetch the inflation rate from a reliable data source
return 2.1;
}
private double GetInterestRate()
{
// Placeholder: Fetch the interest rate from a reliable data source
return 1.2;
}
private double AnalyzeSentiment()
{
// Use ChatGPT to analyze sentiment from news and social media
return _chatGPT.AnalyzeSentiment("Current market news and social media trends");
}
}
// Asset Prediction Agent
public class AssetPredictionAgent
{
private ChatGPTSession _chatGPT;
public AssetPredictionAgent()
{
_chatGPT = new ChatGPTSession();
}
public string PredictAsset(string market)
{
var assets = GetAssets(market);
// Use ChatGPT to evaluate asset performance for selection
var bestAsset = assets.OrderByDescending(a => SimulateAssetPerformance(a)).FirstOrDefault();
return bestAsset?.Name ?? "None";
}
private double SimulateAssetPerformance(Asset asset)
{
// Use ChatGPT to simulate and score the performance of the asset
string response = _chatGPT.Ask($"Simulate performance of {asset.Name} given current market conditions.");
return double.Parse(response); // Parsing response to get performance score
}
private List<Asset> GetAssets(string market)
{
// Example assets list based on market type
return market switch
{
"Crypto" => new List<Asset>
{
new Asset { Name = "BTC" },
new Asset { Name = "ETH" }
},
"Stock" => new List<Asset>
{
new Asset { Name = "AAPL" },
new Asset { Name = "TSLA" }
},
_ => new List<Asset>()
};
}
}
// Asset class for prediction
public class Asset
{
public string Name { get; set; }
}
// Entry Timing Agent
public class EntryTimingAgent
{
private ChatGPTSession _chatGPT;
public EntryTimingAgent()
{
_chatGPT = new ChatGPTSession();
}
public bool ShouldEnterAsset(string asset)
{
// Use ChatGPT to decide the best entry timing
string response = _chatGPT.Ask($"Analyze the current technical indicators and price trends of {asset}. Is it a good time to enter?");
return response.Contains("good time");
}
}
// Monitoring Agent
public class MonitoringAgent
{
private ChatGPTSession _chatGPT;
public MonitoringAgent()
{
_chatGPT = new ChatGPTSession();
}
public void Monitor(string asset)
{
while (true)
{
double currentPrice = GetCurrentPrice(asset);
// Use ChatGPT to provide ongoing analysis
string analysis = _chatGPT.Ask($"Current price of {asset} is {currentPrice}. What is your analysis of the market conditions?");
Console.WriteLine($"AI Analysis: {analysis}");
Thread.Sleep(60000); // Monitor every minute
}
}
private double GetCurrentPrice(string asset)
{
// Placeholder: Fetch current price using an API like Coinbase
return 110; // Example price
}
}
// Decision Agent
public class DecisionAgent
{
private ChatGPTSession _chatGPT;
public DecisionAgent()
{
_chatGPT = new ChatGPTSession();
}
public string MakeDecision(double currentPrice, double buyPrice)
{
// Use ChatGPT to decide the trading action
string query = $"Current price: {currentPrice}, Buy price: {buyPrice}. Should we Buy, Hold, or Sell?";
string aiResponse = _chatGPT.Ask(query);
return aiResponse;
}
}
// Coinbase API class for fetching asset prices - Placeholder implementation
public class CoinbaseAPI
{
private static readonly HttpClient client = new HttpClient();
public async Task<double> GetCryptoPrice(string asset)
{
// Example: Fetch current spot price using Coinbase API
var response = await client.GetAsync($"https://api.coinbase.com/v2/prices/{asset}-USD/spot");
var json = await response.Content.ReadAsStringAsync();
return ParsePrice(json);
}
private double ParsePrice(string json)
{
// Parse the JSON response to extract the price value
var jsonObject = JObject.Parse(json);
return double.Parse(jsonObject["data"]["amount"].ToString());
}
}
// Main Program
class Program
{
static async Task Main(string[] args)
{
// Market selection using ChatGPT
var marketAgent = new MarketSelectionAgent();
var selectedMarket = marketAgent.SelectMarket();
if (selectedMarket == "Out")
{
Console.WriteLine("Staying out of all markets.");
return;
}
// Asset prediction using ChatGPT
var assetAgent = new AssetPredictionAgent();
var selectedAsset = assetAgent.PredictAsset(selectedMarket);
// Entry timing using ChatGPT
var entryAgent = new EntryTimingAgent();
if (entryAgent.ShouldEnterAsset(selectedAsset))
{
// Monitoring and decision-making using ChatGPT
var monitoringAgent = new MonitoringAgent();
var monitoringTask = Task.Run(() => monitoringAgent.Monitor(selectedAsset)); // Run monitoring in parallel
var decisionAgent = new DecisionAgent();
var currentPrice = await new CoinbaseAPI().GetCryptoPrice(selectedAsset);
var decision = decisionAgent.MakeDecision(currentPrice, 100); // Assuming 100 as the buy price
Console.WriteLine($"Decision for {selectedAsset}: {decision}");
}
else
{
Console.WriteLine("Not a good time to enter the market.");
}
}
}
关键变化和增强
- ChatGPT集成:ChatGPTSession类模拟与ChatGPT的交互。在实践中,请使用实际的API调用来替换OpenAI的GPT模型或类似的服务。
- 场景模拟:ChatGPT被用于模拟资产表现,评估市场条件,并决定入场时机。
- 实时决策:ChatGPT提供持续反馈,使交易系统对不断变化的市场动态做出快速响应。
- 并行执行:监控代理作为一个独立任务运行,持续评估市场条件,而其他代理执行其任务。
结论
通过将ChatGPT集成到每个代理人的决策过程中,这个多代理人交易系统变成了一个高度适应性和智能的工具,能够处理复杂的市场动态。ChatGPT能够模拟情景,提供实时分析,并不断从新数据中学习,使其成为优化交易策略的宝贵资产。这种方法为交易提供了一个基于人工智能的新颖框架,利用现代自然语言处理和先进的机器学习模型的全部潜力。