如何计算ChatGPT中的提示成本?
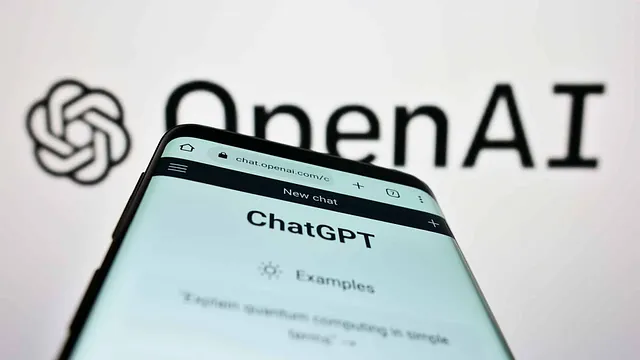
ChatGPT的价格基于发送和接收的令牌数量。要计算交换的总令牌数量,我们可以使用一个叫做tiktoken的库。该库可以解析任何文本并返回其中包含的令牌数量。利用ChatGPT的定价信息,我们可以计算出互动的总价格。
这是方程式。
total_price = (input_tokens * input_price) + (output_tokens * output_price)
import tiktoken
from tiktoken import Encoding
# As of 19th Oct 2023, the price of chat-gpt 3.5 turbo is
input_price = 0.0015 / 1000 # price per 1000 tokens
output_price = 0.002 / 1000 # price per 1000 tokens
def llm_cost_calculator_decorator(llm_function):
"""
A decorator to calculate the input and output cost of Large Language Models like ChatGPT.
This decorator takes llm_function function pointer to pass the prompt and get the response.
:param llm_function: Any Large Language Model which takes prompt as string and gives response as string
:return: Function Pointer
"""
def inner_func(*args):
"""
Calculates the cost of the prompt for a given LLM engine rounded to 4 decimal places
:param args: This is a prompt argument given to llm_function
:return: Returns the response given by the LLM engine
"""
prompt: str = args[0]
prompt_response: str = llm_function(prompt)
enc: Encoding = tiktoken.get_encoding("cl100k_base")
input_token_length: int = len(enc.encode(prompt))
output_token_length: int = len(enc.encode(prompt_response))
content_price: float = input_price * input_token_length
response_price: float = output_price * output_token_length
total_price: float = content_price + response_price
print(f"The cost of the below prompt is: ${round(total_price, 4)}.\n `{prompt}`")
return prompt_response
return inner_func
这个函数是一个装饰器,这意味着它是一个将另一个函数作为参数并返回修改版本的函数。这个装饰器的目的是计算使用像ChatGPT这样的大型语言模型(LLM)的输入和输出成本。ChatGPT是一个能够根据提示生成自然语言回复的人工智能系统。
装饰器接受一个名为llm_function的参数,该参数可以是一个接受字符串作为提示并返回字符串作为响应的任何LLM。装饰器返回一个名为inner_func的函数,该函数接受与LLM函数相同的参数。inner_func执行以下步骤:
- 它将参数分配给名为prompt的变量。
- 它导入一个名为tiktoken的模块,并使用它来获取一个称为enc的编码对象。此对象可以将字符串编码为令牌,这些令牌是L TM可以处理的文本单位。
- 它使用enc.encode方法和len函数来计算提示信息和回答中的令牌数。它将这些数字分配给名为input_token_length和output_token_length的变量。
- 它通过将标记数乘以每个标记的价格来计算提示和响应的成本。每个标记的价格由代码片段开头定义的名为input_price和output_price的变量给出。这些价格以美元为单位,并基于2023年10月19日的日期。它将这些成本分配给名为content_price和response_price的变量。
- 它通过将内容价格和回应价格相加来计算总费用。它将该值赋给一个名为total_price的变量。
- 它使用打印函数和格式化字符串来打印一条显示总成本和提示的消息。
这里有一个使用装饰器的示例代码。
import os
import openai
from decorators import llm_cost_calculator_decorator
@llm_cost_calculator_decorator
def post_content(content: str) -> str:
openai.api_key = os.getenv("OPENAI_API_KEY")
try:
completion = openai.Completion.create(
model="gpt-3.5-turbo",
messages=[
{"content": content}
]
)
message = completion.choices[0].message
print(message)
return message
except Exception as ex:
print(ex)
return ""
if __name__ == "__main__":
print(post_content("what is the capital of France?"))